Question 1: What components define a variable in programming?
- Label, content
- Description, value
- Name, value
- Purpose, type
Question 2: Which of the two following answers are correct?
- Variable names can start with an underscore.
- Variable names can start with a digit.
- Keywords cannot be used as a variable name.
- Variable names can have symbols like: @, #, $, etc.
Question 3: Which of these is not a Python data type?
- int
- string
- decimal
- float
Question 4: What values will the variables x and y hold after the following code has been executed?
x = 10
x += 12
y = x/4
x = x + y
- x will be 27, y will be 5.5
- x will be 27.5, y will be 7
- x will be 27, y will be 5
- x will be 27.5, y will be 5.5
Question 5: How can you change:
print(num)
{‘one’:1,’two’:3)
to
print(num)
{‘one’:1,’two’:2)
- num[2] = ‘two’
- num[1] = ‘two’
- num[‘two’] = 2
- num[‘two’] = ‘2’
Question 6: Which of the following are true of Python lists?
- All elements in a list must be of the same type.
- A list may contain any type of object except another list.
- These represent the same list:[‘a’, ‘b’, ‘c’] [‘c’, ‘a’, ‘b’]
- There is no conceptual limit to the size of a list.
Question 7: a = [‘foo’, ‘bar’, ‘baz’, ‘qux’, ‘quux’, ‘corge’]
Which of the following two codes display correct output?
- print(a[4:-2])
- print(a[-5:-3])
- print(a[-6])
- print(a[1])
Question 8: The code below is for different collections. Select the two code snippets that are correct.
- colors = []
colors. append(‘Red’)
colors. append(‘Green’)
colors. append(‘Yellow’)
print(colors)
- colors = []
colors. insert(‘Red’)
colors. insert(‘Green’)
colors. insert(‘Yellow’)
print(colors)
- frequency = []
frequency [0] = ‘Monday’
frequency [1] = ‘friday’
- frequency = [‘sunday’, ‘monday’, ‘tuesday’,’wednesday’]
frequency. remove(‘monday’)
Question 9: How to return the 5th element from the test list?
- test[5]
- test[4]
- test[‘5’]
- test[‘4’]
Question 10: What is used to concatenate two strings in Python?
- . operator
- + operator
- strcat() function
- ^ operator
Question 11: Which of the following statements are true regarding parameters and return values of a function?
- Parameters of a function are variables defined inside a method signature as being necessary input for performing a task.
- Arguments are values sent to a method.
- Return values are the output, or in other words, the result a function needs to return.
- Parameters and return values are mandatory in functions.
Question 12: What will be the values of the variables x and y by the end of the execution?
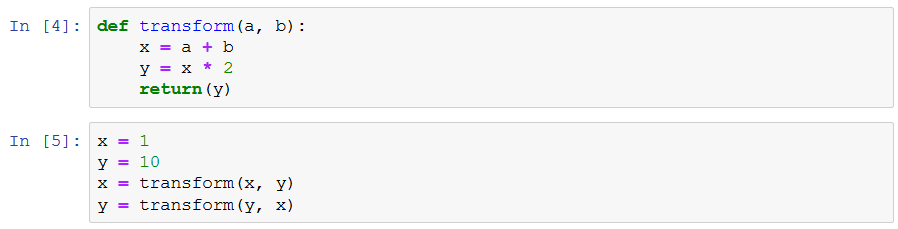
- x -> 1, y -> 10
- x -> 22, y -> 64
- x -> 64, y -> 22
- x -> 1, y -> 64
Question 13: Which of the following statements is true?
- Functions are used to create objects in Python.
- Functions make your program run faster.
- A function is a piece of code that performs a specific task.
- All of the above.
Question 14: Which of the following items must be present in the function header?
- Function name
- Parameter list
- Return value
- def keyword
Question 15: Which the following statements are true regarding parameters and return values of a function?
- Methods are functions that are defined inside a class.
- Methods are functions only for string data types.
- A method can’t change the original object.
- You can call a method with the dot notation: variableName.methodName()
Question 16: a = ‘This is a TEST’
Which of the following codes display the following output:
- print(a.capitalize())
- print(a.upper())
- print(a.lower())
- print(a.firstCapitalize())
Question 17: What is the output of the following snippet of code?
myDict = {‘George’: 4, ‘Jean’: 2, ‘Paul’: 10, ‘Andrew’: 18, ‘Jacob’: 8}
myDict[‘Steve’] = 12
toRemove = [‘George’, ‘Paul’, ‘Jacob’]
for elt in toRemove:
myDict.pop(elt)
print(myDict.values())
- dict_values([2, 10, 18, 8, 12])
- dict_values([2, 10, 8, 12])
- dict_values([2, 18, 12])
- dict_values([12, 18, 2])
Question 18: Suppose you need to print e (exponential) constant defined in the math module. Which of the following code can you use?
- print (math. e)
print(e)
- from math import e
print(e)
- from math import e
print (math. e)
Question 19: Which operator is used in Python to import modules from packages?
- = operator
- _ operator
- operator
- . operator
Question 20:According to the following code, what are the correct outputs?
import numpy as np
myNotes = np.array([0, 5, 10, 15, 20])
- myNotes.mean()
- myNotes[1]
- myNotes.sum()/5
- myNotes.max()